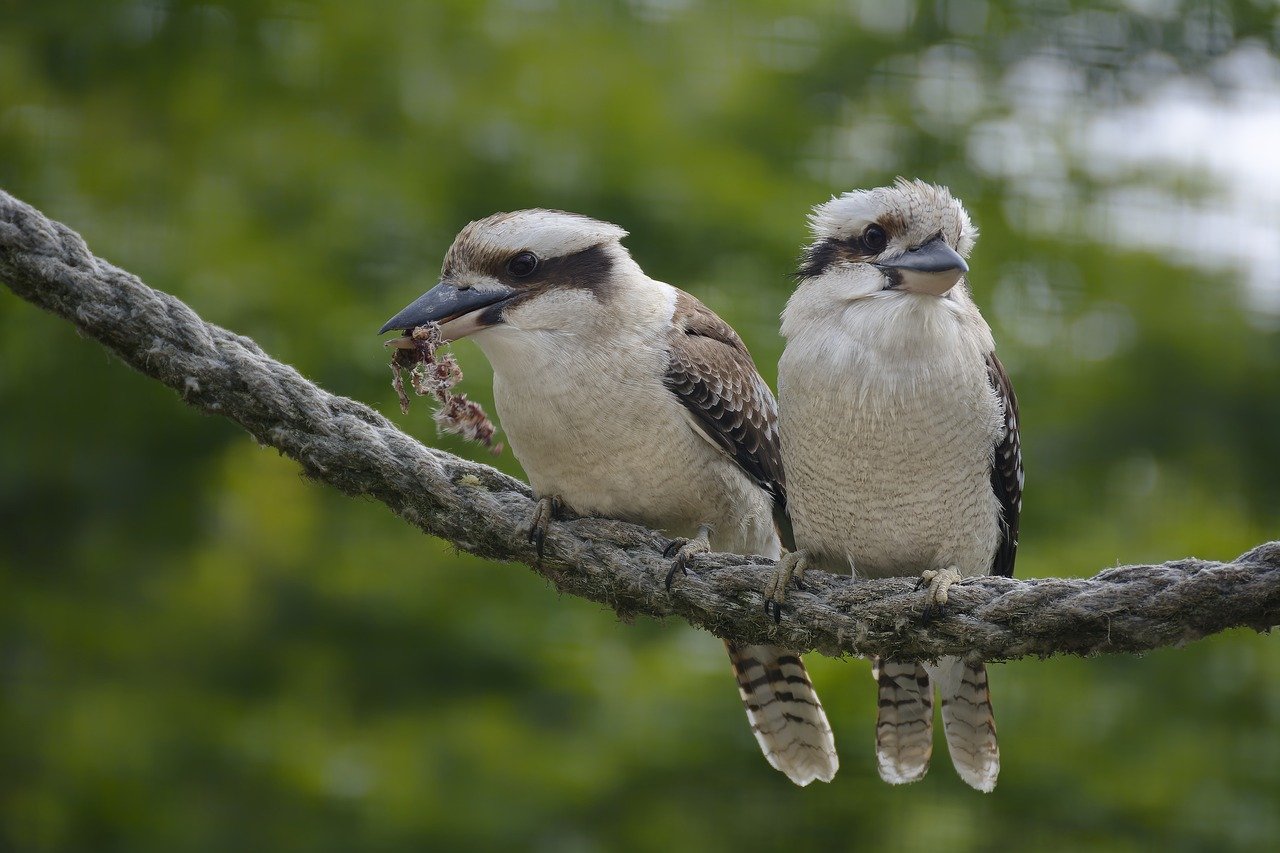
Python中requests
是一个常用的第三方HTTP请求库,可以方便地向网站发送HTTP请求,并获取响应结果。
请注意,requests库不是Python标准库的一部分,你需要使用pip命令来安装它:
pip install requests
另外,可以下载本人编写的接口源码配合示例使用:
点击下载压缩包,压缩包内含有接口源码及使用说明,不需要额外安装requests
关于POST请求
POST请求是一种用于向服务器发送数据,以请求服务器进行处理的方法。
POST请求的特点:
- 数据传输:数据通过请求体发送,不在URL中显示。
- 大小限制:没有严格的大小限制,可以传输大量数据。
- 安全性:比GET请求更安全,因为数据不会显示在URL中。
- 非幂等性:相同的POST请求多次执行可能会有不同的效果,如多次提交表单可能会导致数据重复。
POST请求通常包含请求体,Content-Type 头部非常重要,以下是一些常见的 Content-Type 值及其用途:
-
application/x-www-form-urlencoded:
这是表单数据的标准格式,通常用于 HTML 表单数据的提交。数据被编码为键值对,类似于 URL 查询字符串。
-
multipart/form-data:
用于文件上传和发送包含文件的表单数据。允许你发送二进制数据和文本数据。
-
application/json:
用于发送 JSON 格式的数据。这是 RESTful API 常用的数据格式。
-
text/xml 或 application/xml:
用于发送 XML 格式的数据。现在比较少见,在一些旧的或特定的 API 中仍然使用。
-
text/plain:
用于发送纯文本数据。较少用于 API 请求,除非 API 明确要求。
requests.post()的标准用法
requests.post()
的标准用法用法比较简单,基本用法如下:
requests.post(url,data,json,files,headers=None,cookies,auth,timeout,allow_redirects=True,proxies,hooks,stream=True,verify=True,cert)
其中headers、cookies、auth、timeout、allow_redirects、proxies、hooks、stream、verify、cert
的用法
在使用Requests库发送GET请求中已有说明,本篇不再赘述。
发送 application/x-www-form-urlencoded 数据
将字典传递给 data ,requests 库会自动将字典编码为 application/x-www-form-urlencoded 格式,并设置相应的 Content-Type 头部。
使用 data 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/application/x-www-form-urlencoded/'
# 表单数据
form_data = {
'key1': 'value1',
'key2': 'value2'
}
# 发起 POST 请求
response = requests.post(url, data=form_data)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"application/x-www-form-urlencoded","form":{"key1":"value1","key2":"value2"}},"method":"POST"}
虽然通常不需要手动设置 Content-Type
,因为 requests
会自动处理,但如果你想明确设置,可以这样做:
import requests
url = 'http://www.example.com/api'
form_data = {
'key1': 'value1',
'key2': 'value2'
}
# 明确设置 Content-Type
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.post(url, data=form_data, headers=headers)
print(response.text)
发送 multipart/form-data 数据
将字典传递给 files ,requests 库会自动将数据编码为 multipart/form-data 格式,并设置相应的 Content-Type 头部。
使用 files 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/multipart/form-data/'
# 文件表单数据,文件路径可使用相对路径和绝对路径
files = { # 文件名称 文件路径,这里是相对路径,favicon.png与.py在同一目录下
'file': ('favicon.png', open('favicon.png', 'rb'),
# 文件类型
'image/png'),
'key1': 'value1',
'key2': 'value2'
}
# 发起 POST 请求
response = requests.post(url, files=files)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
favicon.png与.py在同一目录:
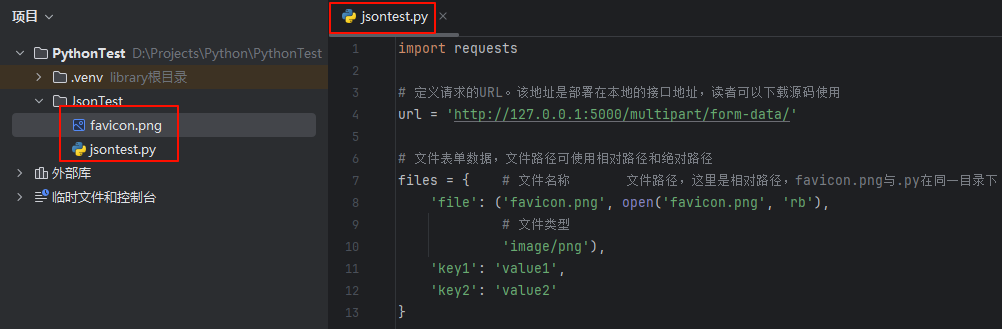
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"multipart/form-data; boundary=6833e61ac38736203cf60c180038b9c6","file_name":"favicon.png","file_type":["image/png",null]},"method":"POST"}
当然你同样可以手动指定 Content-Type
为 multipart/form-data
。
发送 application/json 数据
将字典传递给 json ,requests 库会自动将数据编码为 application/json 格式,并设置相应的 Content-Type 头部。但是有些对象类型,不可以编码,需要事先编码,比如 datatime 对象类型。
使用 json 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/application/json/'
# JSON 数据
json_data = {
'key1': 'value1',
'key2': 'value2'
}
# 发起 POST 请求
response = requests.post(url, json=json_data)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果*
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"application/json","json":{"key1":"value1","key2":"value2"}},"method":"POST"}
使用 data 参数
当字典中有不可编码对象类型时,就需要事先使用别的方法将字典转码为json格式的字符串。需要手动指定 application/json
from datetime import datetime
import requests
import json
# 自定义JSONEncoder类
class CustomEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime):
return obj.isoformat() # 将datetime对象序列化为ISO格式的字符串
return json.JSONEncoder.default(self, obj)
# 定义请求的URL
url = 'http://example.com/api'
# 定义请求体的数据
data = {
'key1': 'value1',
'date': datetime.now()
}
# 将字典转换为JSON格式
json_data = json.dumps(data, cls=CustomEncoder)
# 发送POST请求,headers中指定了请求体的格式为application/json
response = requests.post(url, headers={'Content-Type': 'application/json'}, data=json_data)
# 打印响应的内容
print(response.text)
发送 text/plain 数据
确保发送的数据是纯文本格式。在这种情况下,通常不需要对数据进行编码或序列化。
使用 data 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/text/plain/'
# 纯文本数据
text_data = 'This is plain text data.'
# 自定义请求头
headers = {
'Content-Type': 'text/plain'
}
# 发起 POST 请求
response = requests.post(url, data=text_data, headers=headers)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"text/plain","text":"This is plain text data."},"method":"POST"}
发送 application/xml 或 text/xml
发送 xml 时, Content-Type 定义为 application/xml 或 text/xml 皆可,示例中只演示 application/xml 。
使用 data 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/application/xml/'
# XML 数据
xml_data = """
<note>
<to>Friends</to>
<from>JZY</from>
<heading>Reminder</heading>
<body>Don't forget me!</body>
</note>
"""
# 自定义请求头
headers = {
'Content-Type': 'application/xml'
}
# 发起 POST 请求
response = requests.post(url, data=xml_data, headers=headers)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"application/xml","xml":{"body":"Don't forget me!","from":"JZY","heading":"Reminder","to":"Friends"}},"method":"POST"}
发送 application/octet-stream 数据
打开文件,并以二进制模式读取其内容。然后,使用 data 参数来发送文件的二进制数据,并设置自定义的请求头,将 Content-Type 设置为 application/octet-stream。
使用 data 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/application/octet-stream/'
# 打开文件并读取其二进制内容
with open('favicon.png', 'rb') as file:
# 自定义请求头
headers = {
'Content-Type': 'application/octet-stream'
}
# 发起 POST 请求,发送文件的二进制数据
response = requests.post(url, data=file, headers=headers)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"application/octet-stream","data":"The address of the file : .../PythonApi/data.bin"},"method":"POST"}
按照此方法还可发送 application/pdf、application/zip
等类型数据。application/pdf、application/zip
用来告诉服务端,请求体是什么类型。
发送 application/graphql 数据
定义 GraphQL 查询。然后使用 data 参数发送,并将 Content-Type 设置为 application/graphql 。
使用 data 参数
import requests
# 定义请求的URL。该地址是部署在本地的接口地址,读者可以下载源码使用
url = 'http://127.0.0.1:5000/application/graphql/'
# GraphQL 查询, 查询user表中,id为1的name、email
graphql_query = '''
{
user(id: "1") {
name
email
}
}
'''
# 自定义请求头
headers = {
'Content-Type': 'application/graphql'
}
# 发起 POST 请求,发送 GraphQL 查询
response = requests.post(url, data=graphql_query, headers=headers)
# 打印POST请求的响应的状态码和内容
print('POST请求响应的状态码和内容:')
print(response.status_code)
print(response.text)
运行结果
POST请求响应的状态码和内容:
200
{"blog address":"www.jzy-blogs.cn","message":{"Content-type":"application/graphql","data":{"user":{"email":"JZY@example.com","name":"JZY"}}},"method":"POST"}
END
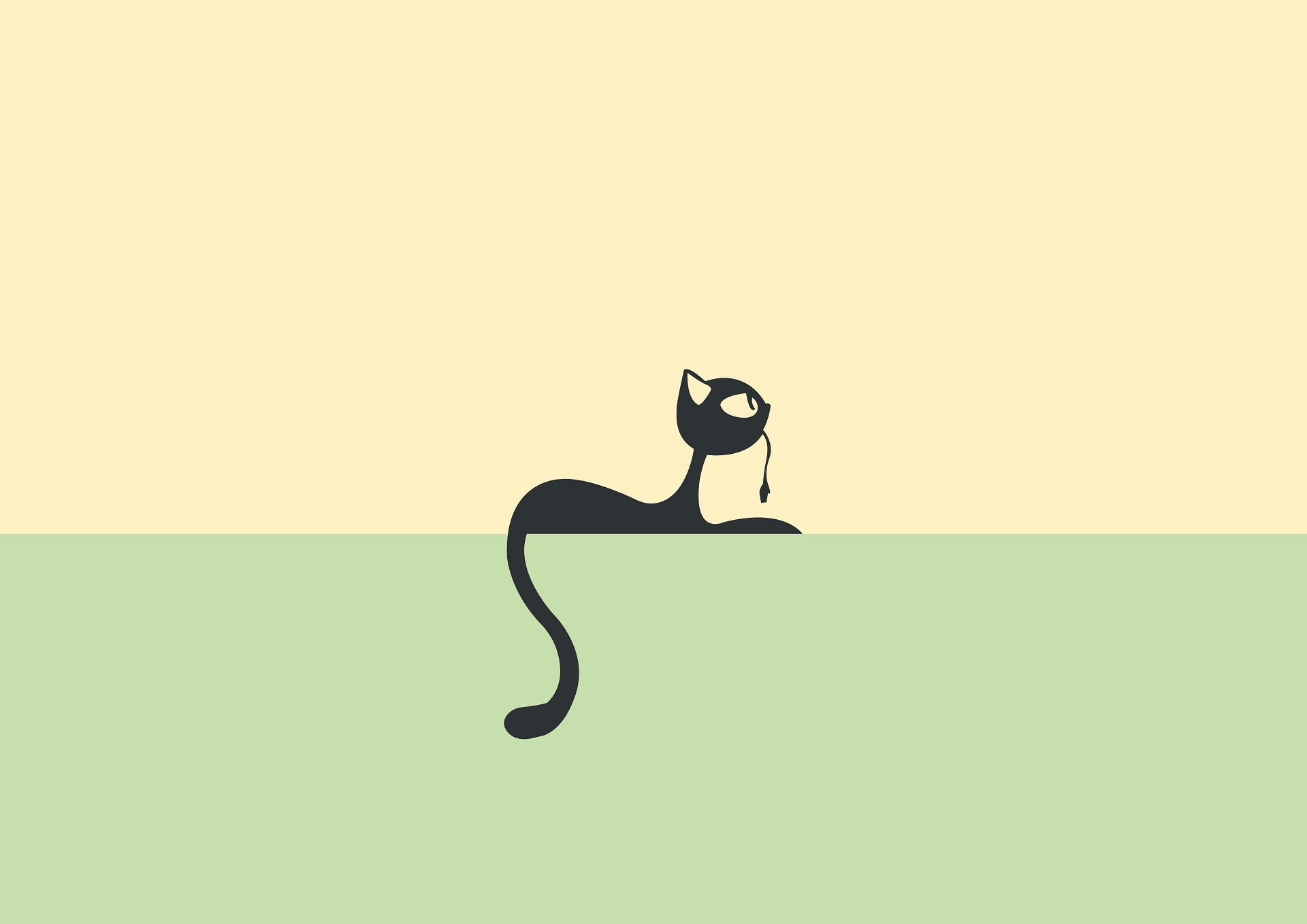
© 转载需要保留原始链接,未经明确许可,禁止商业使用。CC BY-NC-ND 4.0
...